Boolean Operators in Python
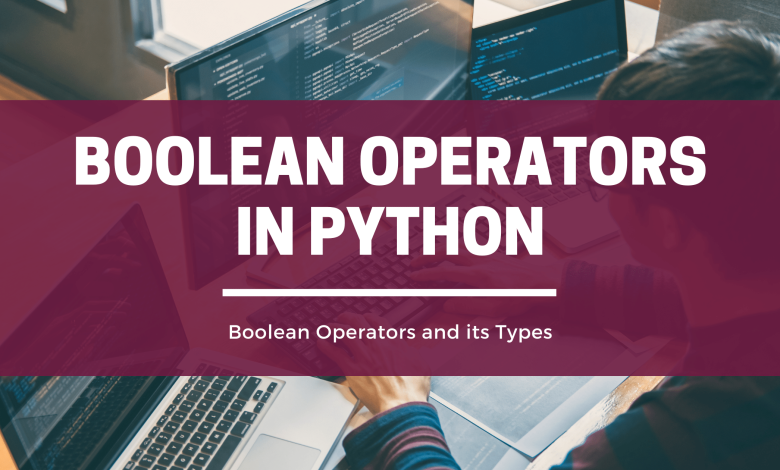
What is the difference between a Boolean Expression and a Boolean Operator?
A boolean expression is one that has only two possible outcomes: true or false. The boolean operators are used when working with multiple boolean expressions or performing some action on them. Because a boolean expression reveals whether it is true or false, operations on these expressions also return “true” or “false.” As a result, there are three different types of boolean operators:
- AND is a conditional operator (&& or “and”)
- The OR operator (|| or “or”) is used to combine two or more variables.
- The NOT operator is used to indicate that something isn’t (not)
AND Boolean Operator in Python
The AND boolean operator works in the same way as the bitwise AND operator, analysing both sides of the expression and returning the result. True and True are synonymous. True and False = False False and True = False False and False = False After that, before we go into the coding, let’s try to grasp this concept in simple English. Let’s say we have the following sentence: I will play if it does not rain today AND there are no extra classes. The results in the above example are dependent on two expressions: There will be no extra classes today if it does not rain. To play, that is, to get a True result, both of the requirements must be true. The AND operator achieves the same thing, except the expressions are conditions instead of variables. If (a 30 and b 45), for instance, Also Read: The Correct Data Governance for Your Organization’s Culture
Non-Boolean Operator.
OR is a bitwise operator that is similar to OR. We were interested in either of the bits being 1 in the bitwise OR. We consider whether or not one of the expressions is true. The result is true if at least one of the expressions is true. True or True = True True or False = True False or True = True False or False = False For example, if it rains today OR there is no extra class, I will play, as in the previous example with the “or” boolean operator. The circumstances are still the same, but the scenario has altered. The introduction of OR means that I will play if it rains, if there is no extra class, or both. I won’t play until it doesn’t rain today and there’s an extra class, in which case both conditions are untrue. We employ conditional expressions with the operator in the programme. If(a > 30 || b <45), for example.
Or Boolean Operator in Python
The NOT operator flips the outcome of the boolean expression that comes after it. It’s important to remember that the NOT operator will only reverse the result of the following expression. Furthermore, the keyword “not” is used to denote the NOT operator. False = not(True) True = not(False) Let’s use an example to try to understand/ comprehend it. If I set a = 30 and b = 30, not((a == b) will evaluate (a == b) first and then reverse the final result, resulting in false. The first (a == b) is calculated if I write the equation as not(a == b) && (c == d). The result is thus reversible due to the NOT operator. The expression (c == d) is then tested. Finally, there is the AND operator. To begin, in a boolean expression, Boolean operators are used to return boolean values. Second, numerous if-else boolean statements can be compressed into a single line of code using Boolean operators.
Conclusion
Finally, there are three types of boolean operators in Python: AND (and) operator OR (or) operator NOT operator